|
Ansoft Designer / Ansys Designer 在线帮助文档:
Script Guide >
Desktop Scripting with IronPython > IronPython Mini-cookbook
IronPython Mini-cookbook
While a tutorial on Python syntax is beyond the scope
of this document, it will present simple counterparts the VBScript constructs
that users tend to regularly use.
Comments
Assigning/Creating variables
Create Lists/Arrays
Create Dictionaries/Maps
Boolean Values
Converting Numbers to
Strings and Vice Versa
String Formatting/Concatenation
Looping over Lists
Looping over a Range
A Note About Indentation
Additional Sections:
Obtaining
More Information
Discovering
Methods
Help on a Method
Comments
VBScript
|
IronPython
|
‘ Comments
start with a single quote
‘ like this line
|
# Comments start
with a sharp or hash
# symbol, like these lines
|
Assigning/Creating Variables
VBScript
|
IronPython
|
‘ Declare
with a Dim
Dim oDesktop
‘Assignment needs a Set instruction
Set oDesktop = oApp.GetAppDesktop()
|
# No Set syntax.
Simply create and assign
oDesktop = oApp.GetAppDesktop()
|
Create Lists/Arrays
VBScript
|
IronPython
|
‘ Declare
as array of String with 11
‘ indices from 0 through 10
Dim myArray(0 to 10) as String
myArray(0) = “Hello”
myArray(1) = “bye”
‘ Declare n array with no size
Dim array2() as String
‘ Re-Dimension the array once size is
‘ known
ReDim array2(0 to 2) as String
array2(0) = “this”
array2(1) = “also”
|
# Declare an
empty array
myEmptyArray = []
# declare an array and initialize it with 5 ints
myInitedArray = [ 1, 2, 3, 4, 5]
# Python lists can have items of any type
# and there is no pre-declaration
# declare an array and init with mixed types
mixed = [“hello”, 1 ,2 [“nested”]]
# append to an array
mixed.append( 3.5 )
|
Create Dictionaries/Maps
VBScript
|
IronPython
|
‘ No direct
equivalent is available as
‘ far as the author knows
|
# an IronPython
dictionary is a collection of
# name value pairs. Just like arrays, there is
# no restriction on the keys or the values.
# For purposes of Ansoft scripting however,
# all keys must be strings
# delimiters are curly braces
# use a “:” between the key and the
value
# separate key value pairs with a “,”
myDict = {
“a” : 1,
“b” : “hello there”,
“c” : [ 1, 2, “abc”]
}
|
Boolean Values
VBScript
|
IronPython
|
‘ Boolean
literals are in lower case
true
false
|
# The first letter
is capitalized
True
False
|
Converting Numbers to Strings and Vice Versa
VBScript
|
IronPython
|
‘ Use CInt,
CDbl, CBool, CLng
‘ to convert the string representation
‘ to the number representation. Use
‘ IsNumber to check before conversion
Dim nStr = “100”
Dim n = CInt(nStr)
‘ Use CStr to convert a number to
‘ its string representation
Dim v, vStr
v = 100
vStr = CStr(v)
|
# use the integer()
or float() or double()
# functions to cast a string CONTAINING the
# string representation of whatever you are
# casting to.
strInt = “3”
intVal = int(strVal)
floatVal = float(strVal)
# invoke the str() function with the int/float
# values as needed. You can alternately use
# the string formatting method listed below
strVal = str(42)
strVal = str(42.345)
|
String formatting/concatenation
VBScript
|
IronPython
|
‘ string
concatenation uses the &
‘ operator
Dim allStr, str1
str1 = “ how are you”
allStr = “Hello “ & “ There”
& str1
‘ there seems to be no direct string
‘ formatting function in VBScript
‘ using string concatenation or using
‘ Replace are the two builtin options
Dim fmt = “{1} climbs stalk {2}”
Dim str = Replace(fmt, “{1}”, “jack”)
str = Replace(str, “{2”}, 10)
|
# if you have
two strings, you can always
# concatenate then using the ‘+’ operator
str1 = “hello”
str2 = “world”
str12 = str1 + “ “ + str2
# if you have different types though, string
# and int say, you must use the string
# formatting commands. When formatting
# multiple arguments, they must be entered
# as a tuple ( item1, item2, )
num = 10
str3 = “%s climbs stalk %d” % (“jack”,
num)
str4 = “%d stalks” % num
|
Looping over lists
VBScript
|
IronPython
|
Dim myArray(0
to 2) as String
myArray(0) = “alpha”
myArray(1) = “bravo”
myArray(2) = “charlie”
For Each i in myArray
Print i
Next
|
vals = [1, 3,
3.456]
def process(val):
return 2*val
# is of the form
# for variable_name in array ‘:’
# < indent> statement1
# <indent> statement2
for i in vals:
print i
print “ -> ” process(i)
|
Looping over a range
VBScript
|
IronPython
|
‘ Loop
over a range, specify start, end
‘ and step
For i = 0 To 10 Step 1
Print i
Next
|
# prints out
values from 0 through 9
for i in range(0, 10):
print i
|
A note about indentation
Python is one of the thankfully rare languages where
whitespace (spaces, tabs etc) are syntactically significant. Whatever
your feelings on the subject, you must understand the basics of indentation
before scripting in python.
Any statement that introduces a block of code should
be written such that every line of the block has the same indent (leading
spaces or tabs) and the indent should be at least one more than the
indent of the introducing statement.
# define a function that starts at 0 indentation.
def multInt(a,b):
# every line following the def multInt which is
expected to
# be a part of the function, must have the indent
used by the
# first line of the function (3 spaces)
# here we introduce one more block, the if
condition
# each line that belongs to the body of this func
should have
# an indent that is more than the indent used
by the if
# statement
If a%2 == 0:
# I am using an indent 2 more than the parent.
i.e. 5
# spaces in total
return (a * b) + 100
else:
return (a * b) + 1000
Sample Script 1: Describing python indentation
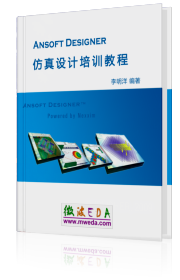
HFSS视频教程
ADS视频教程
CST视频教程
Ansoft Designer 中文教程
|
|